How to End a Program in Python
If you want to know how to end a program in Python, you’ve come to the right place. Ending a program is an important skill for any programmer. Sometimes, programs need to stop running. This could be because they finished what they needed to do. Other times, it could be due to an error.
In this article, we will explore different ways to exit a program in Python. We will also look at why it is important to know how to terminate a Python program.
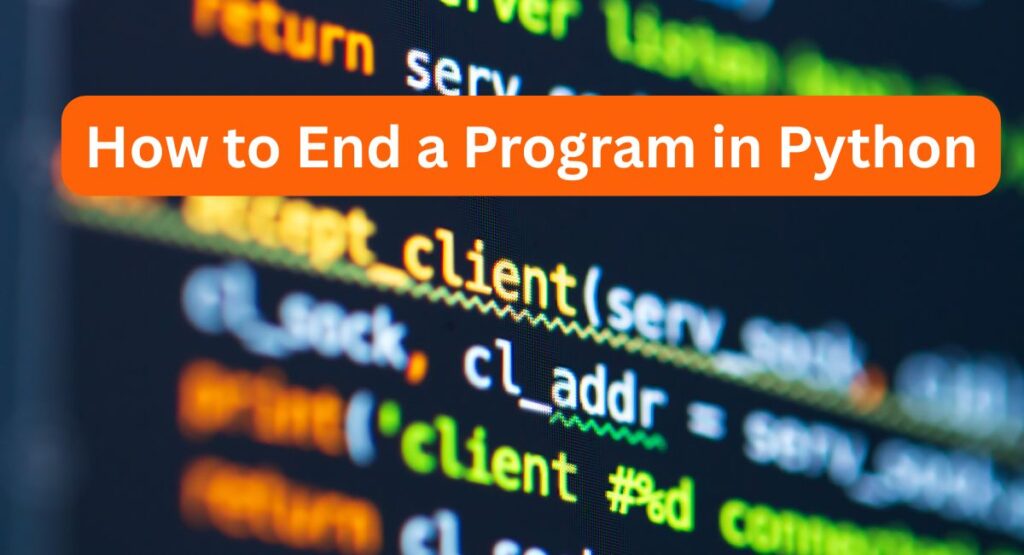
Read more >>> How to Upgrade Python Version: A Simple Guide
Why Do We Need to End Programs?
Every program has a start and an end. Programs can run for a short time or a long time. Sometimes, they finish quickly, and other times, they take longer. Knowing how to exit a program in Python is useful for many reasons:
- Completing Tasks: After running a task, you want the program to finish.
- Handling Errors: If something goes wrong, you may need to stop the program.
- Freeing Resources: When a program ends, it frees up memory and other resources.
Basic Ways to End a Program
Using the exit()
Function
One of the simplest ways to end a program in Python is by using the exit()
function. You can type this in your code:
exit()
When Python reaches this line, the program stops running. This is a quick and easy way to end your program.
Using the sys.exit()
Function
Another way to exit a program is to use the sys.exit()
function. First, you need to import the sys
module at the top of your code:
import sys
Then, you can call the function like this:
sys.exit()
This method is often used in larger programs. It works similarly to exit()
, but gives you more options.
Read more >>> A Comprehensive Beginner’s Guide on Logarithmic Python
Using a Return Statement
If you are inside a function, you can use the return
statement to end that function. When the function ends, the program continues. But if the function is the main part of your program, it can stop the entire program.
Here’s a simple example:
def main():
print("Hello, World!")
return
main()
In this case, after the print statement, the program will stop because the function has ended.
Stopping a Program Due to an Error
Sometimes, you may want to end a program because of an error. You can do this with the raise
statement. This lets you create a custom error. Here’s how to do it:
raise Exception("An error occurred!")
When Python sees this line, it will stop running the program and show the error message.
Using Keyboard Interrupt
If you are running a program in a terminal or command line, you can stop it with a keyboard interrupt. This is usually done by pressing Ctrl + C
. This will immediately stop the program. It’s a handy way to quickly exit a Python program.
Read more >>> How to Square in Python
Conclusion
Knowing how to end a program in Python is very important. Whether you use exit()
, sys.exit()
, or other methods, it helps you control your program. You can stop it when it’s done or if something goes wrong.
In this article, we learned different ways to exit Python programs. We also discussed why it is essential to know how to terminate a Python program. By understanding these concepts, you can become a better programmer.
So, the next time you write a Python program, remember these tips. You will be able to manage your programs effectively, making your coding journey even better!